Writing Tests
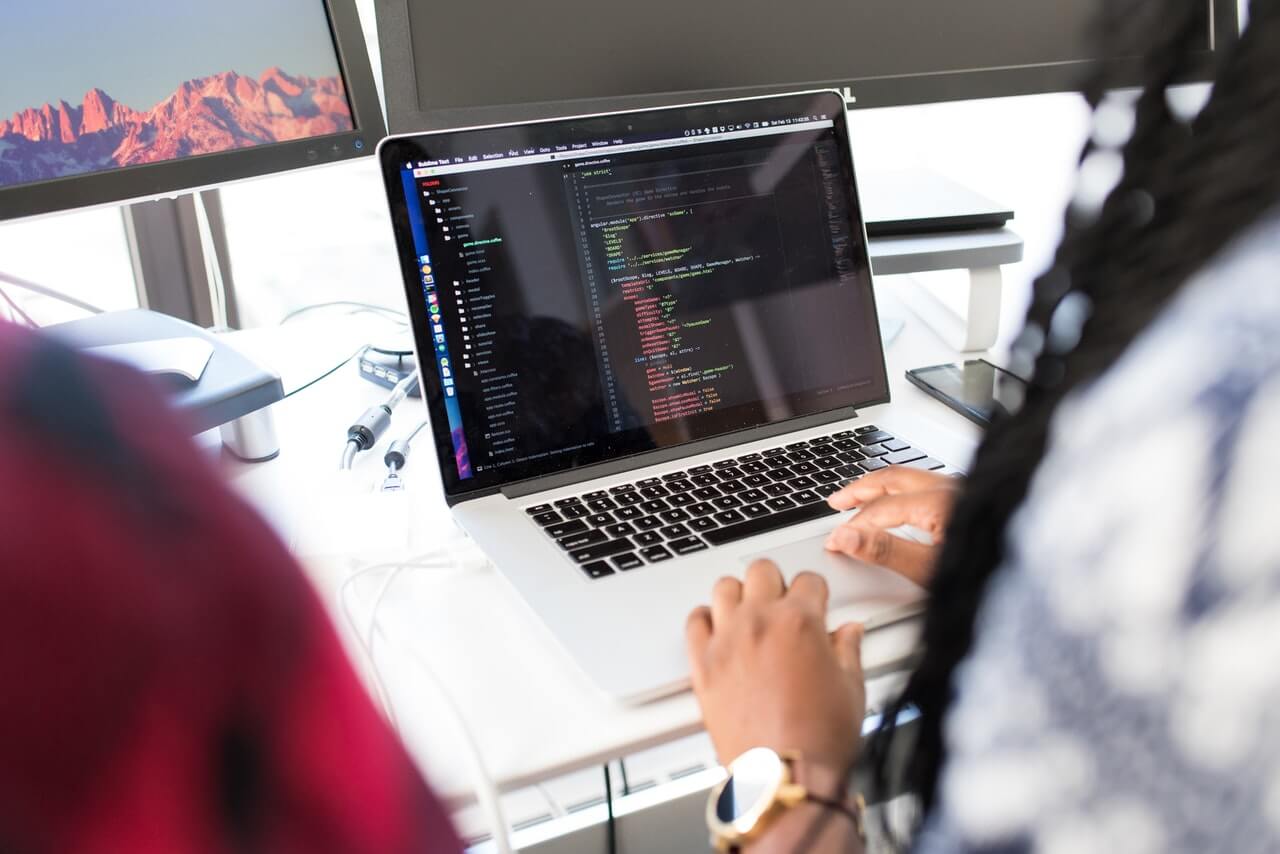
PhpUnit
- PHPUnit is a unit testing framework for the PHP programming language. It is an instance of the xUnit architecture for unit testing frameworks that originated with SUnit and became popular with JUnit.
Mocha
- Mocha is a JavaScript test framework for Node.js programs, featuring browser support, asynchronous testing, test coverage reports, and use of any assertion library.
Jest
- Jest is a JavaScript testing framework maintained by Meta, designed and built by Christoph Nakazawa with a focus on simplicity and support for large web applications. It works with projects using Babel, TypeScript, Node.js, React, Angular, Vue.js and Svelte.
Reasons to test
- Helps check whether an application behaves as expected
- Safeguard against unwanted behaviour when changes are made
- Automated process that is efficient on the long term.
What to test
- High value features.
- Edge cases in high value features.
- THings that are easy to break.
- Basic React component testing (User interactions, conditional rendering, utils/hooks)
Laravel testing
- To perform testing you can run the command php artisan test.
- Each test file in the tests/ folder is a PHP Class extending the TestCase of PHPUnit. Inside of each class, you may create multiple methods, usually one method for one situation to be tested. Inside of each method, there are three actions: preparation of the situation, then action, and then checking (asserting) if the result is as expected.
- php artisan make:test ExampleTest generate a feature test
- Laravel Breeze comes with tests inside
- use Illuminate\Foundation\Testing\RefreshDatabase; is required if testing actions may affect the database. For that, one would need to create a separate testing database that would be refreshed with php artisan migrate:fresh, every time the tests are executed.
Backup the Database before testing to avoid loss of data after migrate:refresh
Feature tests try to run the actual features of your applications: get the URL, call the API, mimic the exact behavior like filling in the form. Feature tests usually execute the same or similar thing as any project user would do, manually, in real life.
php artisan make:test OrderPriceTest --unit to generate a unit test
Unit tests process is about testing a specific non-public unit of your code, in isolation. For example, you have some Laravel class with a method that calculates something, like the total price of the order with parameters. So, your unit test would assert if the correct results are returned by that method (unit of code), with different parameters. Unit tests don't touch the database, they work like a black box, in isolation from the running application
Unit testing in react
- screen.debug() // console logs the body
Examples of simple integration script
```javascript // The test below follows the Arrange, act, assert pattern // Arrange - rendering component // act - performing actions, userEvents // assert - make assertions to check conditions
import { render, screen } from "@testing-library/react"; import userEvent from "@testing-library/user-event"; import TransactionCreateStepTwo from "./TransactionCreateStepTwo";
test('if an amount and note is entered, the pay button becomes enabled', async () => { render(<TransactionCreateStepTwo sender={{ id: '5' }} receiver={{ id: '5' }} />);
// screen.debug() console logs the body
expect(await screen.findByRole('button', { name: /pay/i })).toBeDisabled();
userEvent.type(screen.getByPlaceholderText(/amount/i), "50");
userEvent.type(screen.getByPlaceholderText(/add a note/i), "dinner");
expect(await screen.findByRole('button', { name: /pay/i })).toBeEnabled();
});
- Async await uses findByRole, normally getByRole works. Use async await if there's a delay in rednering or loading of components
- In integration tests, check the realistic user flows
- Cypress only works with cy.findBy
- Cypress use a test-id (data-test) for dynamic fields e.g. cy.get("[data-test=sidenav-user-balance]").then($balance => oldBalance = $balance.text());