Home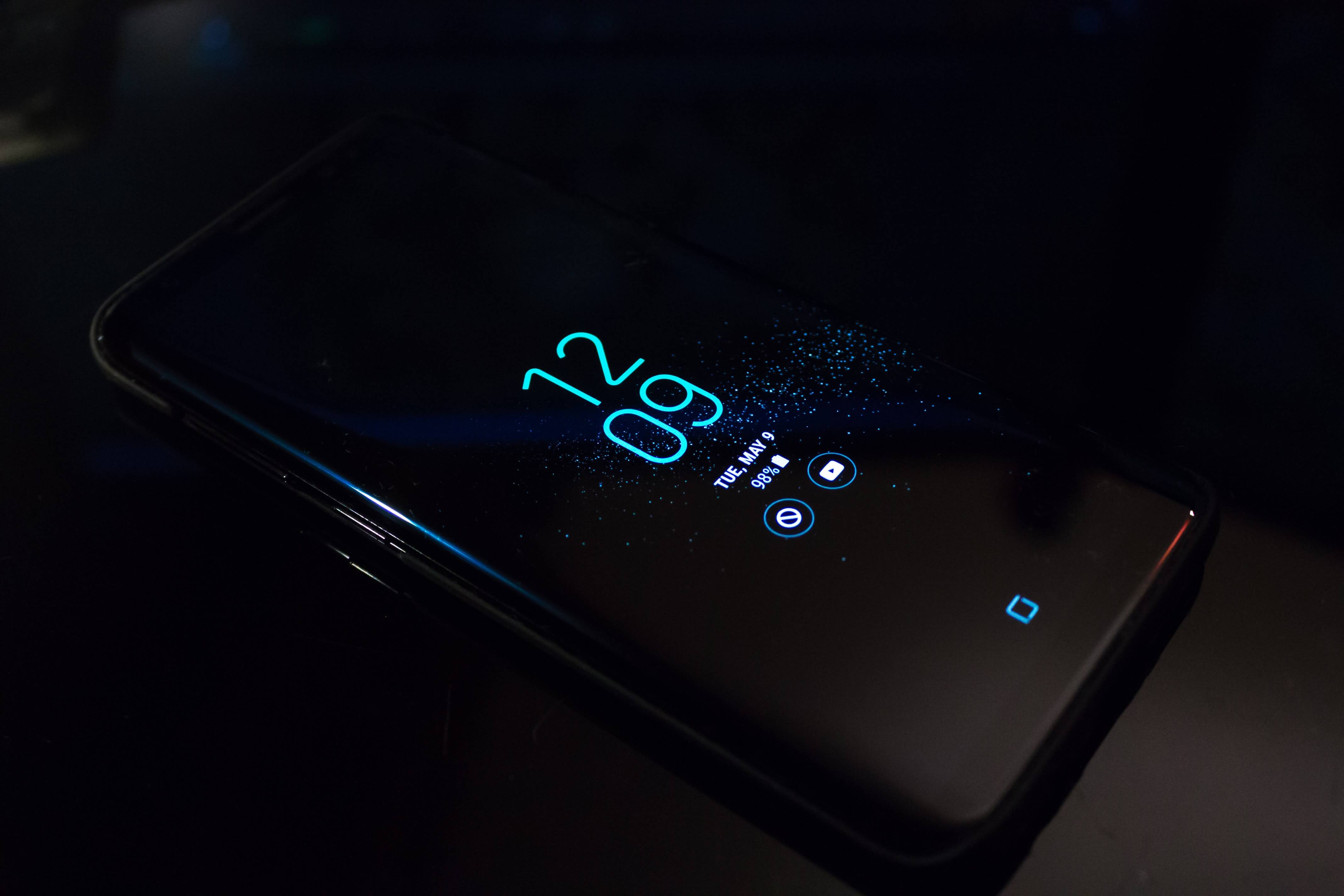
React Native
Posted on Feb 01, 2023
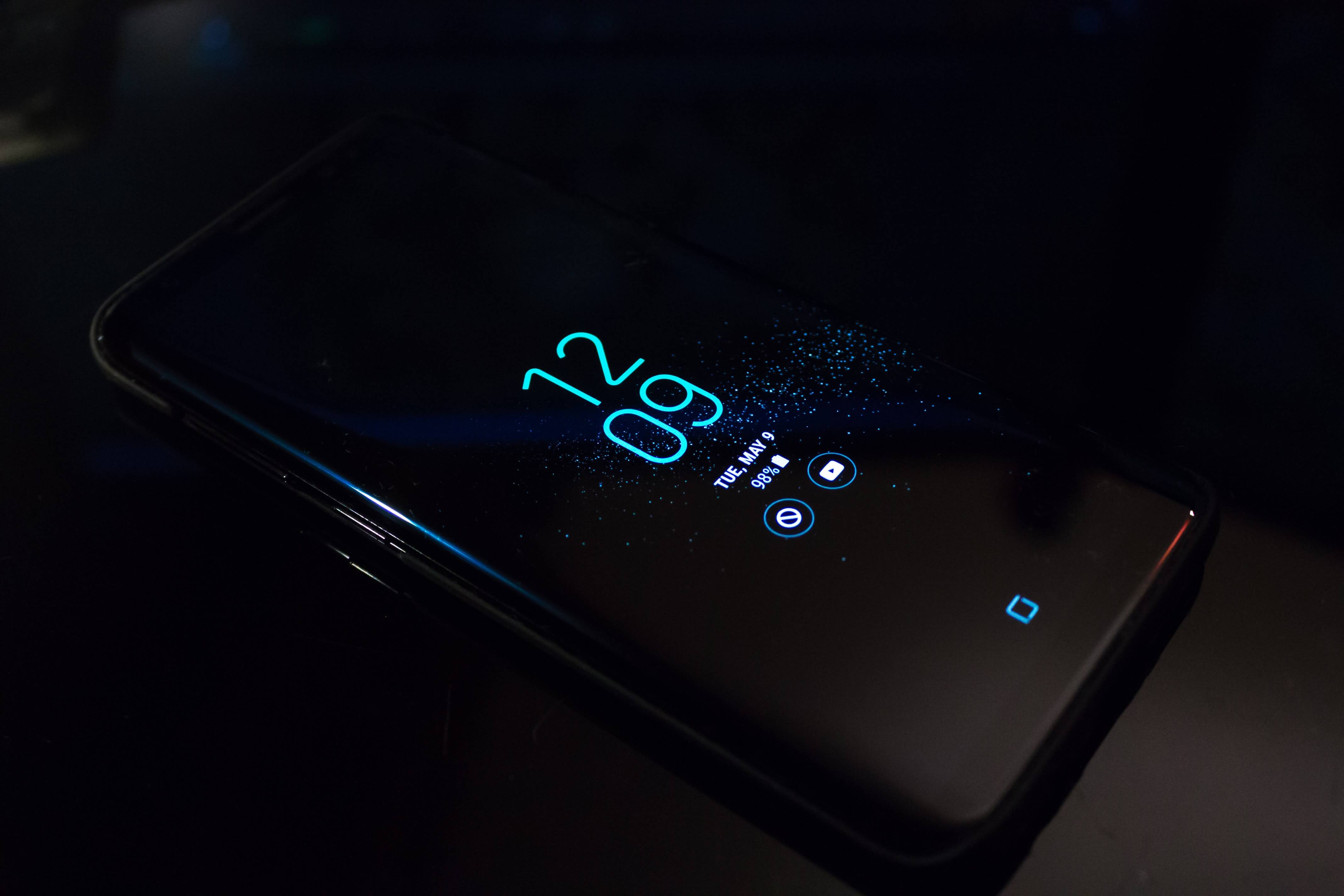
React Native
- Used to create native apps for Android, iOS, and more using React by combining the best parts of native development with React, a best-in-class JavaScript library for building user interfaces. It lets you create truly native apps and doesn't compromise your users' experiences. It provides a core set of platform agnostic native components like View, Text, and Image that map directly to the platform’s native UI building blocks.
Tailwind and react Native
- NativeWind, tailwin-rn twrnc packages help create tailwind components
- A react native View component is similar to a non-scrolling div, Text to a p, ScrollView(can hold multiple views and multiple components) to a div.
- State is like a component’s personal data storage, it is useful for handling data that changes over time or that comes from user interaction, it gives your components memory.
- Props are arguments you use to configure how components render.
// Setting styles
const styles = StyleSheet.create({
container: {
flex: 1,
paddingTop: 22,
},
item: {
padding: 10,
fontSize: 18,
height: 44,
},
});
<View style={styles.container>
Flatlist
- FlatList works well for long lists of data, where the number of items might change over time. Unlike the more generic ScrollView, the FlatList only renders elements that are currently showing on the screen, not all the elements at once.
e.g.
<FlatList data={[ {key: 'Devin'}, {key: 'Dan'}, {key: 'Dominic'}, {key: 'Jackson'}, {key: 'James'}, {key: 'Joel'}, {key: 'John'}, {key: 'Jillian'}, {key: 'Jimmy'}, {key: 'Julie'}, ]} renderItem={({item}) => <Text style={styles.item}>{item.key}</Text>} />
SectionList
Render a set of data broken into logical sections, maybe with section headers. e.g.
<SectionList sections={[ {title: 'D', data: ['Devin', 'Dan', 'Dominic']}, { title: 'J', data: [ 'Jackson', 'James', 'Jillian', 'Jimmy', 'Joel', 'John', 'Julie', ], }, ]} renderItem={({item}) => <Text style={styles.item}>{item}</Text>} renderSectionHeader={({section}) => ( <Text style={styles.sectionHeader}>{section.title}</Text> )} keyExtractor={item => `basicListEntry-${item}`} />
Checking OS platform ```javscript import {Platform, StyleSheet} from 'react-native';
const styles = StyleSheet.create({ height: Platform.OS === 'ios' ? 200 : 100, });
```
Pressable component
- A core component wrapper that can detect various stages of interactions, from basic single-tap events to advanced events such as a long press.
expo-image-picker library
- Provides access to the system's UI to select images and videos from the phone's library or take a photo with the camera.
Modal component
- That presents content above the rest of your app.
react-native-view-shot library
- Allows taking a screenshot
expo-media-library
- Allows accessing a device's media library to save an image.
dom-to-image
- Third-party library to capture a screenshot on the web and save it as an image.
Splash screen
- A screen that is visible before the contents of the app has had a chance to load. It hides once the app is ready for use and the content is ready to be displayed.