React Crash Course
Posted on March 8, 2021
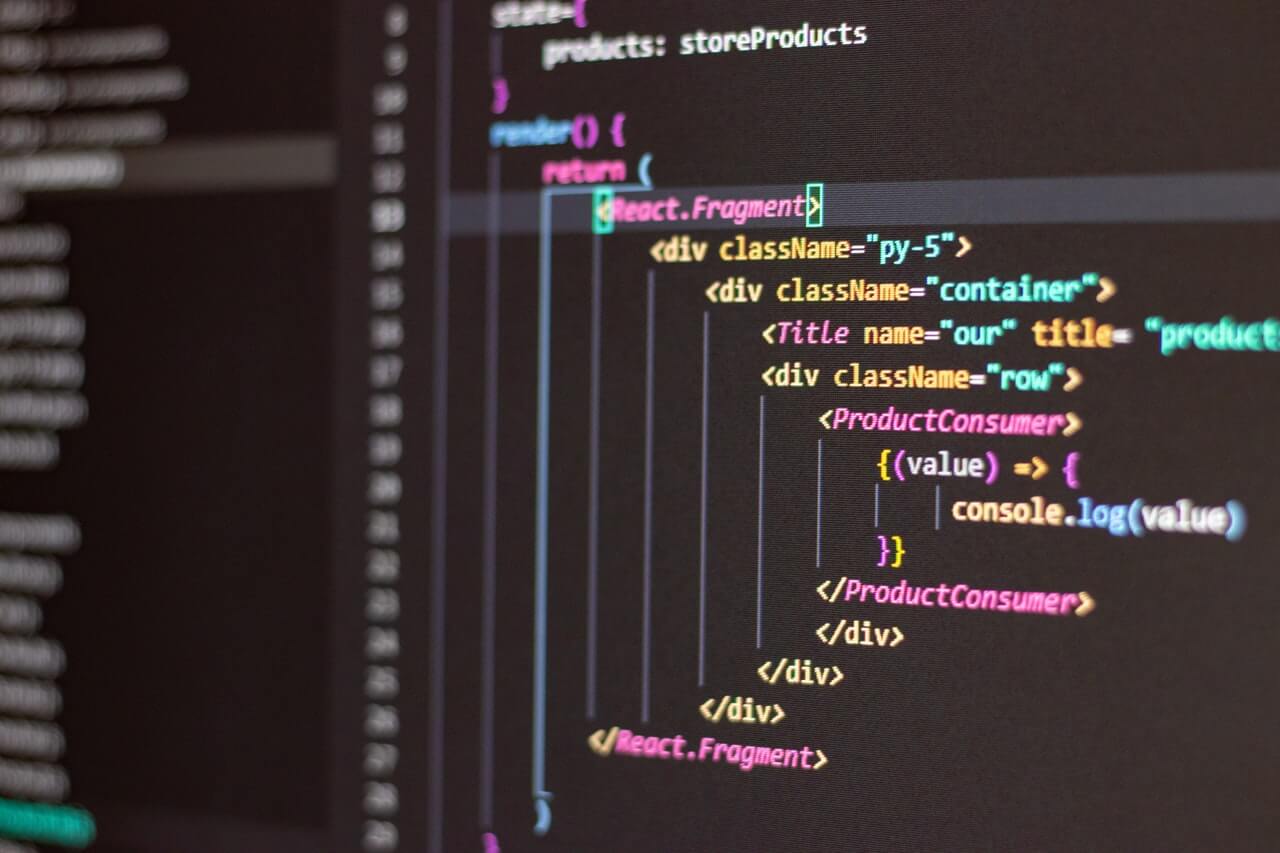
A declarative, efficient, and flexible JavaScript library for building user interfaces.
React. js is an open-source JavaScript library that is used for building user interfaces specifically for single-page applications. It's used for handling the view layer for web and mobile apps. React also allows us to create reusable UI components.
React is a free and open-source front-end JavaScript library for building user interfaces based on UI components. It is maintained by Meta and a community of individual developers and companies.
In a controlled component, form data is handled by a React component. The alternative is uncontrolled components, where form data is handled by the DOM itself.
To write an uncontrolled component, instead of writing an event handler for every state update, you can use a ref to get form values from the DOM.
Development tips
// Use react query to fetch data and not useEffect
const { status, data, error, isFetching } = useQuery(
['data'],
async () => {
const data = await (
await fetch(`${API_BASE_URL}/data`)
).json()
return data
}
)
React.Strictmode - StrictMode is a tool for highlighting potential problems in an application. Like Fragment, StrictMode does not render any visible UI. It activates additional checks and warnings for its descendants. StrictMode currently helps with:
- Identifying components with unsafe lifecycles
- Warning about legacy string ref API usage
- Warning about deprecated findDOMNode usage
- Detecting unexpected side effects
- Detecting legacy context API
- Ensuring reusable state
Short-circuit evaluation.
{condition && <ConditionalComponent />}
I.e., if the first operand (condition) is falsy, the AND operator (&&) stops and it does not evaluate the second operand (
- Reason why eslint issues a warning when you use a function inside an array, the function is re-allocated on render because it's within render scope, and therefore the function reference changes between renders, causing your useEffect to every time (based on shallow comparison of the dep array), so useCallback avoids that and keeps the reference the same between renders.
// Linting problem when a function is called in an array, a hack
const fetchNextUser = useRef(() => {});
fetchNextUser.current = () => {logic};
useEffect(() => { fetchNextUser.current() }, []);
- useContext hook, work with context api, share data without passing props
const moods = { happy: 'happy', sad: 'sad'}
const MoodContext = createContext(moods);
const App = (props) => {
return (
<MoodContext.Provider value={moods.happy}>
<MoodEmoji />
</MoodContext.Provider>)
}
const MoodEmoji = () => {
const mood = useContext(MoodContext); // Consume value from nearest parent provider
return <p>{ mood }</p>
}
- useReducer, hook that is used to handle reactive data using a redux pattern
const App = () => {
function reducer(state, action) {
switch (action.type) {
case 'increment':
return state + 1;
case 'decrement':
return state - 1;
default:
throw new Error();
}
}
const [state, dispatch] = useReducer(reducer, 0);
return (
<>
Count : {state}
<button onClick={() => dispatch({type: 'decrement'})}>-</button>
<button onClick={() => dispatch({type: 'increment'})}>+</button>
</>)}
- Babel: the name of the compiler used to take JSX and turn it into createElement calls
- Avoid copying the values of props into a component's state because you want to allow a component to update in response to changes in the props
- Children prop - a special property that JSX creates on components that contain both an opening tag and a closing tag, referencing it's contents.
- Virtual DOM - a representation of a user interface that is kept in memory and is synced with the "real" DOM is called what?
- Error boundaries - React component that catches JavaScript errors anywhere in the child component tree.
- Use instead of since triggers a full page reload.
- Reconciliation, process of deciding whether an update is necessary.
- Webpack - used to split your app into smaller chunks that can be more easily loaded by the browser.
- webpack command: runs react local development server
- Flux keeps the data uni-directional