Node js?
Posted on July 5, 2021
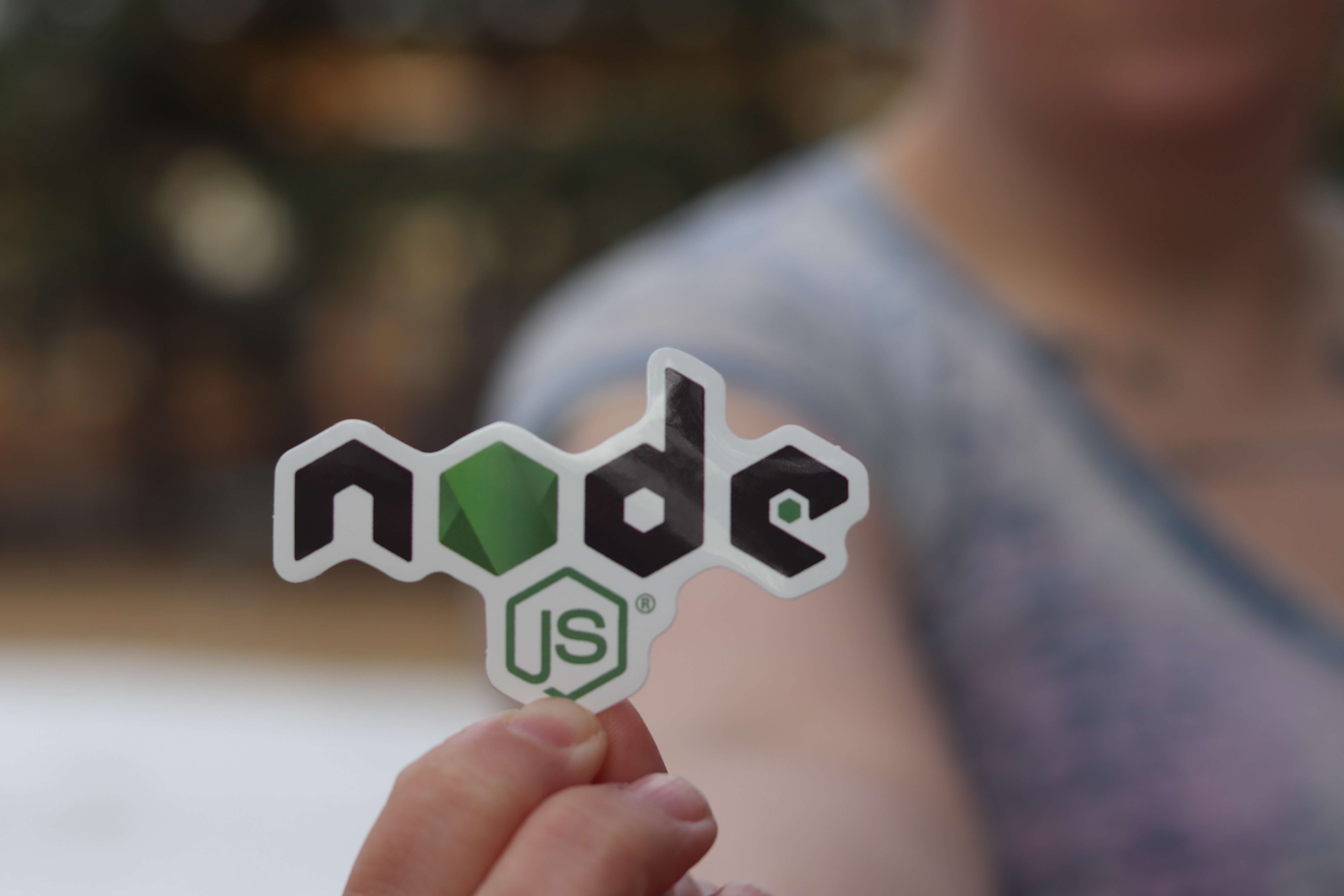
Nodejs
A JavaScript runtime that allows you to run js on a server. It is a single-threaded event loop-based architecture. It executes code in a single sequence or direction. Asynchronous and Single-Threaded: Execution doesn't wait for the current request to complete and moves to the next request/call. It extends the power of handling file and network I/O with a preference for asynchronous patterns after including everything that is Javascript.
Chrome’s V8 often called an engine, is a package deal containing an interpreter, compiler, runtime implementation compatible with the runtime interface demanded by the ECMA standard JavaScript conventions.
Fast, efficient and highly scalable. Event driven, non-blocking I/O model, asynchronous...works on a single thread using non-blocking I/O calls. It supports tens of thousands concurrent connections. Optimizes throughput & scalabilty in apps with many I/O calls, (PHP is synchronous so it runs on multiple threads so requests spawn on new threads and in the end much memory is taken, has to wait for one process to load) Not good to use with CPU intensive apps(heavy calculations) Single thread supports concurrency via events & callbacks Node.js is very good at one thing, and that is saturating an I/O pipe. It is also single-threaded, which makes it really good at saturating just one core on a multi-core CPU
Good for REST API & Microservies Real time services(chat, live updates) CRUD Apps - blogs, shopping carts, social networks Tools and utilities
Node JS Platform does not follow Request/Response Multi-Threaded Stateless Model. It follows Single Threaded with Event Loop Model.
The language itself is single-threaded, but the browser APIs act as separate threads. The browser APIs are built into the browser. Based on the command received from the call stack, the API starts its own single-threaded operation.
Each request will still get the same amount of CPU time as multithreaded applications but Node doesn’t need to start a thread. A single-threaded app fails big if you need to do lots of CPU calculations before returning the data. Things like doing Fourier transform (mp3 encoding for example), ray tracing (3D rendering), etc.
Single Threaded Event Loop Model Processing Steps:
- Clients Send request to Web Server.
- Node JS Web Server internally maintains a Limited Thread pool to provide services to the Client Requests.
- Node JS Web Server receives those requests and places them into a Queue. It is known as “Event Queue”.
- Node JS Web Server internally has a Component, known as “Event Loop” which uses indefinite loop to receive requests and process them.
- Event Loop uses Single Thread only. It is main heart of Node JS Platform Processing Model.
- Even Loop checks any Client Request is placed in Event Queue. If no, then wait for incoming requests indefinitely. If yes then pick up one Client Request from Event Queue
- Starts process that Client Request
- If that Client Request Does Not requires any Blocking IO Operations, then process everything, prepare response and send it back to client.
- If that Client Request requires some Blocking IO Operations like interacting with Database, File System, External Services then it will follow different approach
- Checks Threads availability from Internal Thread Pool
- Picks up one Thread and assign this Client Request to that thread.
- That Thread is responsible for taking that request, process it, perform Blocking IO operations, prepare response and send it back to the Event Loop
- Event Loop in turn, sends that Response to the respective Client.
NodeJS as a waiter taking the customer's orders while the I/O chefs prepare them in the kitchen. Other systems have multiple chefs, who take a customer's order, prepare the meal, clear the table and only then attend to the next customer.
Benefits of single thread approach
No overhead when spawning new threads.
Your code is much easier to reason about, as you don’t have to worry about what will happen if two threads access the same variable because that simply cannot happen.
- Write multiple async/await functions instead of one big async/await is because what you are writing is blocking code inside the async function
Tips
- Any time you see a function that ends in Sync, it blocks i.e. it will need to work before any of the other code can run e.g.
const { readFile, readFileSync } = require('fs');
const txt = readFileSync('./file.txt','utf8');
To make it non blocking, refactor it to a callback can be used,
const { readFile } = require('fs');
readFile('./hello.txt','utf8', (err, txt) => {console.log(txt)});
So Node registers the callback, excecutes the rest of the script then finally runs the callback when the file has been read
A 3rd way is to use promises which are async and non-blocking so
const { readFile } = require('fs');
const file = await readFile('./hello.txt','utf8')
Express js
A JavaScript back-end framework that's designed to develop complete web applications and APIs. Express is the back-end component of the MEAN stack, which also includes MongoDB for the database, AngularJS for the front end and Node
Javascript modules
//person.js
class Person {
constructor(name, age) {
this.name = name;
this.age = page;
}
greeting() {
console.log(`${this.name} and ${this.age}`);
}
module.exports = Person
}
//index.js
const Person = require("./person");
const person1 = new Person('Collins',24);
person1.greeting();
Javascript functions
const os = require('os');
const fs = require('fs');
// Platform
os.platform();
// CPU Arch
os.arch();
// CPU Core Info
os.cpus();
// Free memory
os.freemem();
// Total memory
os.totalmem();
// Home dir
os.homedir();
// Uptime
os.uptime();
const path = require('path');
// File paths
// Base file name
path.basename(__filename);
// Directory name
path.dirname(__filename);
// File extension
path.extname(__filename);
// Create path object
path.parse(__filename).base);
// Concatenate paths
path.join(__dirname, 'test', 'hello.html');
// get the path delimiter base on the current OS Environment
const platSpec = path.delimiter;
// Create folder
fs.mkdir(path.join(__dirname, '/test'), {}, err => {
if (err) throw err;
console.log('Folder created...');
});
// Create and write to file
fs.writeFile(
path.join(__dirname, '/test', 'hello.txt'),
'Hello World!',
err => {
if (err) throw err;
console.log('File written to...');
// File append
fs.appendFile(
path.join(__dirname, '/test', 'hello.txt'),
' I love Node.js',
err => {
if (err) throw err;
console.log('File written to...');
}
);
}
);
// Read file
fs.readFile(path.join(__dirname, '/test', 'hello.txt'), 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
// Rename file
fs.rename(
path.join(__dirname, '/test', 'hello.txt'),
path.join(__dirname, '/test', 'helloworld.txt'),
err => {
if (err) throw err;
console.log('File renamed...');
}
);
const URL = require('url').URL;
// Serialized query
console.log(myUrl.search);
// Params object
console.log(myUrl.searchParams);
err.code = ENONET //page not found
// Debug node application
// In package json in scripts, add
"start" : "node --inspect script.js"