Laravel Tips?
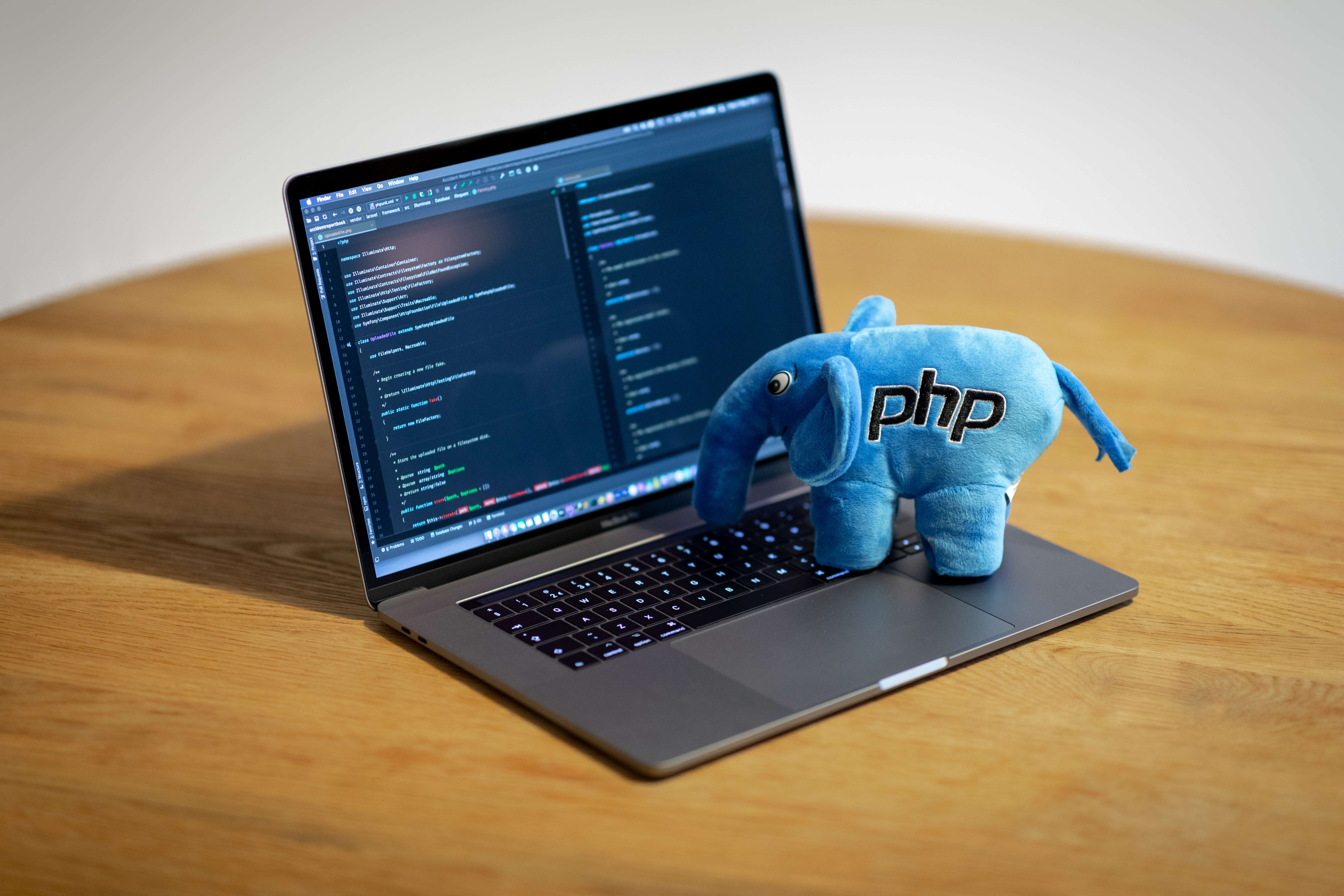
Unauthenticated HTTP status code will be: 401 Unauthorised HTTP status code will be: In Laravel, there are two ways to authorize a user. One approach is closure based, another approach is class-based. Closure based approach is called Gates. The class-based approach is called policy.
Gates are most applicable to actions which are not related to any model or resource, such as viewing an administrator dashboard. In contrast, policies should be used when you wish to authorize an action for a particular model or resource.
Modifying column attributes
Schema::table('users', function (Blueprint $table) {
$table->string('name', 50)->change();
});
Modifying column names
Schema::table('users', function (Blueprint $table) {
$table->string('name', 50)->nullable()->change();
});
Renaming columns
Schema::table('users', function (Blueprint $table) {
$table->renameColumn('from', 'to');
});
php artisan optimize:clear // clear cache
Dropping columns
$table->dropColumn('description');
Creating dummy data
php artisan tinker
Model::factory()->count(5)->create()
// Handling many to many on blade
<ul>
@foreach($order->products as $item)
<li>{{ $item->name }} ({{ $item->pivot->quantity }} x
${{ $item->selling_price }})</li>
@endforeach
</ul>
// Creating
<tbody class="bg-white border-none">
@foreach (old('products', ['']) as $index => $oldProduct)
<tr id="product{{ $index }}">
<td class="px-4 py-3 text-sm">
<select id="products" name="products[]" class="">
<option value="">-- choose product --</option>
@foreach ($products as $product)
<option value="{{ $product->id }}"
{{ $oldProduct == $product->id ? ' selected' : '' }}>
{{ $product->name }}
</option>
@endforeach
</select>
</td>
<td class="px-4 py-3 text-sm">
<x-input type="number" id="quantity"
name="quantities[]" min="1" class="block w-full"
value="{{ old('quantities.' . $index) ?? '1' }}" required />
@error('quantity')
<span class="text-xs text-red-600 dark:text-red-400">
{{ $message }}
</span>
@enderror
</td>
</tr>
@endforeach
<tr id="product{{ count(old('products', [''])) }}"></tr>
</tbody>
@section(scripts)
<script>
$(document).ready(function(){
let row_number = {{ count(old('products', [''])) }};
$("#add_row").click(function(e){
e.preventDefault();
let new_row_number = row_number - 1;
$('#product' + row_number).html($('#product' +
new_row_number).html()).find('td:first-child');
$('#products_table').append('<tr id="product' + (row_number + 1) + '"></tr>');
row_number++;
});
$("#delete_row").click(function(e){
e.preventDefault();
if(row_number > 1){
$("#product" + (row_number - 1)).html('');
row_number--;
}
});
});
</script>
@endsection
// Storing data
public function store(Request $request)
{
$products = $request->input('products', []);
$quantities = $request->input('quantities', []);
$sum =0;
for ($product = 0; $product < count($products); $product++) {
$price = Product::where(['id'=>$products[$product]])->first()->selling_price;
$sum += $price * $quantities[$product];
}
$order = Order::create(
[
"order_status" => $request->order_status,
"sold_by" => $request->sold_by,
"payment_means" => $request->payment_means,
"pre_tax_total" => $sum
]
);
for ($product = 0; $product < count($products); $product++) {
if ($products[$product] != '') {
$order->products()->attach($products[$product],
['quantity' => $quantities[$product]]);
}
}
return back();
}
/**
* Get the user that owns the phone.
*/
public function user()
{
return $this->belongsTo(User::class, 'foreign_key', 'owner_key');
}
Services and Dependency Injections
Sometimes we need to put application logic somewhere outside of Controllers or Models, it’s usually are so-called Services. But there are a few ways to use them – as static “helpers”, as objects, or with Dependency Injection.
Different techniques to move code from Controller to Service:
*First Way: From Controller to Static Service “Helper” *Second Way: Create Service Object with Non-Static Method *Third Way: Service Object with a Parameter *Fourth Way: Dependency Injection – The Simple Case *Fifth Way: Dependency Injection with Interface – Advanced Usage
// Creating a foreign key
$table->index('user_id');
$table->foreign('user_id')->references('id')->on('users')->onDelete('cascade');
// On drop
$table->dropForeign('lists_user_id_foreign');
$table->dropIndex('lists_user_id_index');
$table->dropColumn('user_id');
// Option 2
$table->foreignId('user_id')->constrained('parent_table_name')->cascadeOnDelete();
// Saving data even after delete
$table->foreignId('user_id');
Class Post {
public function user () {
return $this->belongsTo(User::class)->withDefault(['name' => 'Ghost user']);
}
}
Determine table constraints
SELECT TABLE_NAME,COLUMN_NAME,CONSTRAINT_NAME, REFERENCED_TABLE_NAME,REFERENCED_COLUMN_NAME FROM INFORMATION_SCHEMA.KEY_COLUMN_USAGE WHERE REFERENCED_TABLE_SCHEMA = 'mjengo_coreapp' AND REFERENCED_TABLE_NAME = 'requisition_schedules';
php artisan vendor:publish --provider="" // make the package configurations public by copying the configuration file from the vendor to config/<file>.php
Attribute casting
- provides functionality similar to accessors and mutators without requiring you to define any additional methods on your model. Instead, your model's $casts property provides a convenient method of converting attributes to common data types. The $casts property should be an array where the key is the name of the attribute being cast and the value is the type you wish to cast the column to.
protected $casts = [ 'status' => TaskStatus::class ];
Tips
- Laravel throws 404 on servers is if the rewrite mode is turned off. Solution: Enable rewrite on server
Composer error
- Use --ignore-platform-req=ext-posix --ignore-platform-req=ext-pcntl when installing