Django Crash Course
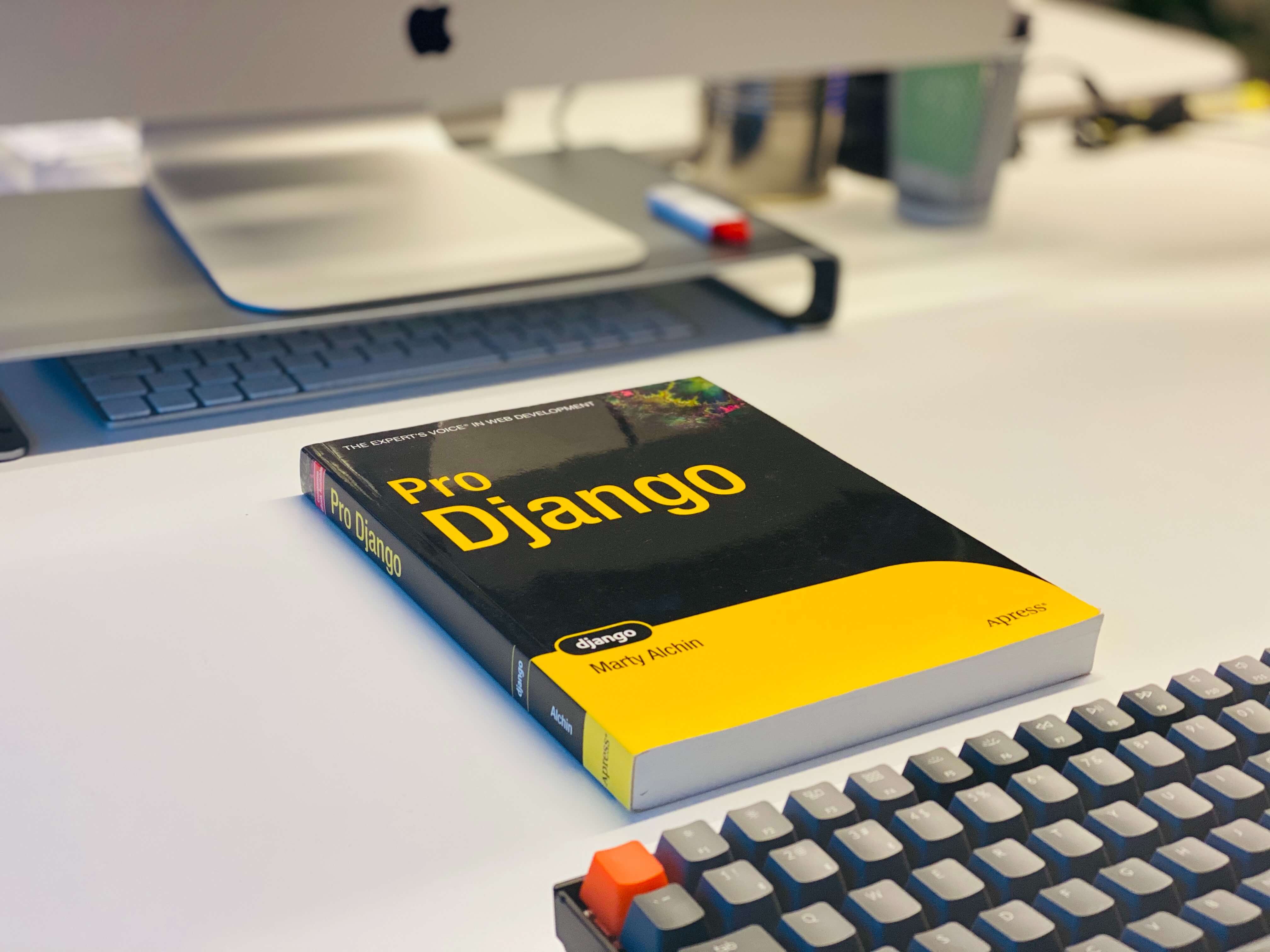
Django is a fully featured high-level web application framework with loads of features. It's a Python web framework that can be used to build complex web applications. One of the great things about the Django framework is its in-depth documentation.
A Django website consists of a single project that is split into separate apps. The idea is that each app handles a self-contained function that the site needs to perform. The Django project holds some configurations that apply to the project as a whole, such as project settings, URLs, shared templates and static files. Each application can have its own database and has its own functions to control how the data is displayed to the user in HTML templates.
Each application also has its own URLs as well as its own HTML templates and static files, such as JavaScript and CSS. Django apps are structured so that there is a separation of logic. It supports the Model-View-Controller Pattern, which is the architecture on which most web frameworks are built.
The basic principle is that in each application there are three separate files that handle the three main pieces of logic separately:
Model defines the data structure. This is usually a database and is the base layer to an application.
View displays some or all of the data to the user with HTML and CSS.
Controller handles how the database and the view interact.
Django handles the controller part itself.
The pattern Django utilizes is called the Model-View-Template (MVT) pattern. The view and template in the MVT pattern make up the view in the MVC pattern. All you need to do is add some URL configurations to map the views to, and Django handles the rest!
A Django site starts off as a project and is built up with a number of applications that each handle separate functionality. Each app follows the Model-View-Template pattern.
Django, you don’t need to learn a new language because it has a built-in Object Relational Mapper (ORM).
An ORM is a program that allows you to create classes that correspond to database tables. Class attributes correspond to columns, and instances of the classes correspond to rows in the database. When you’re using an ORM, the classes you build that represent database tables are referred to as models.
To create instances of our Project class, we’re going to have to use the Django shell. The Django shell is similar to the Python shell but allows you to access the database and create entries. To access the Django shell, we use another Django management command:
Setting up a new django project, in conda prompt:
- mkdir development_folder
- cd development_folder
- python -m venv venv
- venv\Scripts\activate
- pip install Django
- django-admin startproject project_name
- move project folders up to make them top level
- python manage.py runserver
- python manage.py startapp app_name
- open http://127.0.0.1:8000/ in the browser
Project Structure
- init.py tells Python to treat the directory as a Python package.
- admin.py contains settings for the Django admin pages.
- apps.py contains settings for the application configuration.
- models.py contains a series of classes that Django’s ORM converts to database tables.
- tests.py contains test classes.
- views.py contains functions and classes that handle what data is displayed in the HTML templates.
Useful commands
python manage.py shell
python manage.py createsuperuser
pip install -r requirements.txt
py manage.py tailwind start # run development server for tailwind
python -m smtpd -n -c DebuggingServer localhost:1025
# create a local smtp server that can be used to confirm email and such
python manage.py showmigrations # show mmigrations
python manage.py migrate --fake core zero # clear migration history
pip3 freeze > requirements.txt #generate requirements.txt file
General steps
Setup prject
Setup app
Create Views in view.py
Map views to url by creating a urls.py file
Register routes in urls file
Register routes in project folder
python manage.py collectstatic - Gathering static files in a single directory so you can serve them easily.
Models
- Setup DB
- Create model
- Migrate python manage.py makemigrations then python manage.py migrate